Use PowerShell to Create and Manage Users and Groups in SharePoint Online
I have an upcoming talk at TechEd 2014 and part of it will be spent showing you how you can manage users with PowerShell in SharePoint Online. I thought, I would give a little preview and show you some of the ways you can manage users. If you are using Office 365, this post will get you started, creating, deleting, and adding users to groups. You'll also learn how to set permissions on groups as well as promote site collection administrators. You'll need to install SharePoint Online Management Shell, if you haven't already.Get started by opening a session using Connect-SPOService and the full URL to the admin site of your tenant. You can see my first SharePoint Online PowerShell post for the syntax.
You'll need to know the URL to the site collection you are working with for all of these commands.
Getting a list of users
We can start by getting a simple list of all users on a site using Get-SPOUser.Get-SPOUser -Site https://mytenant.sharepoint.com/sites/mysitecollection
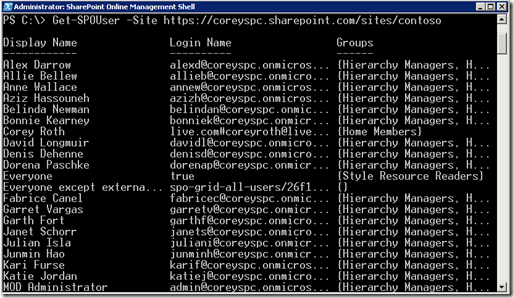
This will give you a list of all users, the login name, and what groups each user belongs to. If you look all the way down the list, you'll even notice some internal hidden users such as the cache, crawl, and system accounts.
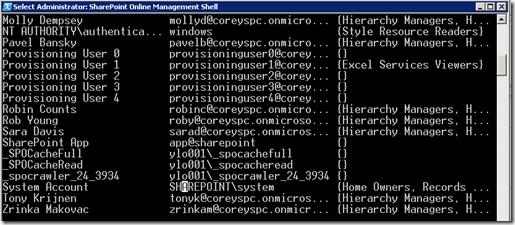
Even with a small list, you'll notice that this cmdlet takes a while to execute. You can filter it by specifying a specific user or group. For example, to retrieve the user information for our user, Sara Davis, we use the same cmdlet with the -LoginName parameter. Keep in mind you have to specify the full login name.
Get-SPOUser -Site https://mytenant.sharepoint.com/sites/mysitecollection -LoginName user@mytenant.onmicrosoft.com

To view all of the users in a group, use the -Group parameter.
Get-SPOUser -Site https://mytenant.sharepoint.com/sites/mysitecollection - Group "My Group"
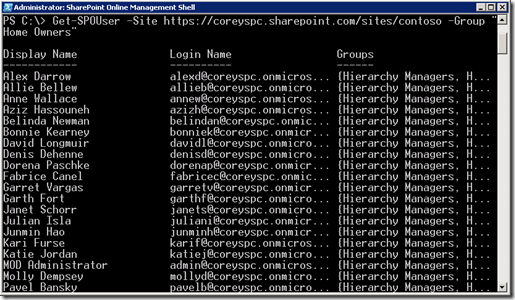
You'll notice there are not any commands to add new users. Those are handles at the Office 365 level. If the user requires a brand new account, you create the user there and then add them to the appropriate groups.
Getting a list of groups
We can retrieve a list of groups on a given site using Get-SPOSiteGroup.Get-SPOSiteGroup -SiteName https://mytenant.sharepoint.com/sites/mysitecollection
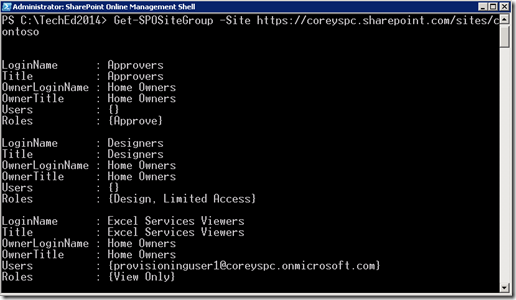
This will show you the name of the group, the roles of the group, and what users are in it. You may want to use FormatTable to make the results easier to read.
Get-SPOSiteGroup -SiteName https://mytenant.sharepoint.com/sites/mysitecollection | FT Title, Roles -AutoSize
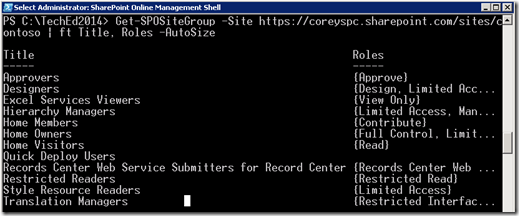
You can also request a specific group by name, with the -Group parameter
Get-SPOSiteGroup -SiteName https://mytenant.sharepoint.com/sites/mysitecollection -Group "My Group"
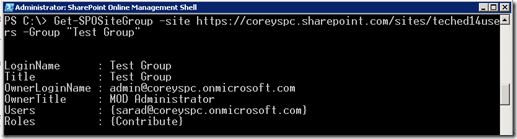
Creating a group
To create a Group, we use the New-SPOSiteGroup cmdlet. You will need to pass the name of the group using the -Group parameter as well as the site collection. In the -PermissionLevels attribute, you pass the name of a known permission level such as Contribute, Design, or Full Control.New-SPOSiteGroup -Site https://mytenant.sharepoint.com/sites/mysitecollection -Group "Group Name" -PermissionLevels "Contribute"
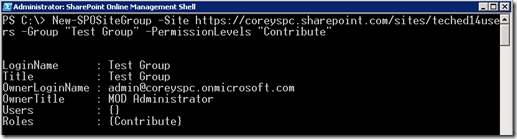
This cmdlet tends to take a while. Once it's done, it will return information about your group. If you assign the return value of this cmdlet to a variable, you can then pass it to Add-SPOUser to add a user to the group.
Adding a user to a group
Once you create a group, you will probably want to add a user to it. We can do that with Add-SPOUser.Add-SPOUser -Site https://mytenant.sharepoint.com/sites/mysitecollection -Group "Group Name" -LoginName user@mytenant.onmicrosoft.com

Using Get-SPOUser, like we showed earlier, we can verify our new users is in the group.

Removing a user from a group
As you might guess, removing a user from a group takes the same three parameters with the Remove-SPOUser cmdlet. However, the Group is optional. Include it to remove the user from a specific group or omit it to remove the user from the site entirely.Remove-SPOUser -Site https://mytenant.sharepoint.com/sites/mysitecollection -Group "Group Name" -LoginName user@mytenant.onmicrosoft.com

When successful, no output will be returned.
Adding a permission level to a group
To change permissions on a group, we use the Set-SPOSiteGroup To add a permission level to a group, use the -PermissionLevelsToAdd parameter. Note this cmdlet uses the -Identity parameter instead of -Group.Set-SPOSiteGroup SPOUser -Site https://mytenant.sharepoint.com/sites/mysitecollection -Identity "Group Name" -PermissionLevelsToAdd "Design"
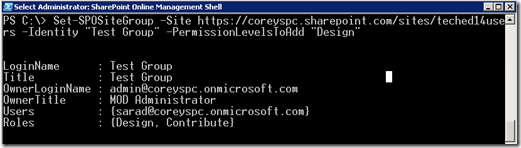
Removing a permission level from a group
We can also use Set-SPOSiteGroup to remove a permission level as well using the -PermissionLevelsToRemove parameter.Set-SPOSiteGroup SPOUser -Site https://mytenant.sharepoint.com/sites/mysitecollection -Identity "Group Name" -PermissionLevelsToRemove "Contribute"
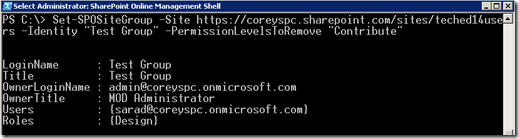
You can also use Set-SPOSiteGroup to rename it with the -Name parameter as well as change the owner with the -Owner parameter.
Removing a group
To remove the group, use the Remove-SPOSiteGroup. For some reason, this cmdlet uses the -Identity parameter instead of -Group so pass the name there.Remove-SPOSiteGroup SPOUser -Site https://mytenant.sharepoint.com/sites/mysitecollection -Identity "Group Name"

Make a user a site collection administrator
We can give users site collection administrator rights, but the functionality is buried in Set-SPOUser. Set the -IsSiteCollectionAdmin command to $true to make the user a site collection administrator.Set-SPOUser -Site https://mytenant.sharepoint.com/sites/mysitecollection -Group "Group Name" -LoginName user@mytenant.onmicrosoft.com -IsSiteCollectionAdmin $true

To remove site collection administrator rights, simple set IsSiteCollectionAdmin to $false.
Get a list of site collection administrators
If you want to see who all of the site collection administrators are, you can find the value on the IsSiteAdmin property of the user object returned from Get-SPOUser. You just have to display it. In the example below, we select the column using Format-Table (ft).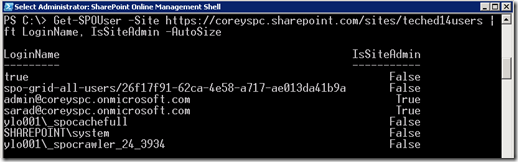
We'll be covering this and a lot more at my PowerShell talk at TechEd next week. If you are there, be sure and attend.
Using PowerShell to Manage SharePoint 2013 Online
Getting Started
Before you can start working with the SharePoint Online cmdlets you must first download those cmdlets. Having the cmdlets as a separate download (separate from SharePoint on-premises that is) allows you to use any machine to run the cmdlets. All we have to do is make sure we have PowerShell V3 installed along with the .NET Framework v4 or better (required by PowerShell V3). With these prerequisites in place simply download and install the cmdlets from Microsoft: http://www.microsoft.com/en-us/download/details.aspx?id=35588.Once installed open the SharePoint Online Management Shell by clicking Start > All Programs > SharePoint Online Management Shell > SharePoint Online Management Shell. Just like with the SharePoint Management Shell for on-premises deployments the SharePoint Online Management Shell is just a standard PowerShell window. You can see this by looking at the target attribute of the shortcut properties:
C:\Windows\System32\WindowsPowerShell\v1.0\powershell.exe -NoExit -Command "Import-Module Microsoft.Online.SharePoint.PowerShell -DisableNameChecking;"
As you can see from the shortcut, a PowerShell module is loaded: Microsoft.Online.SharePoint.PowerShell. Unlike with SharePoint on-premises, this is not a snap-in but a module, which is basically the new, better way of loading cmdlets. The nice thing about this is that, like with the snap-in, you can load the module in any PowerShell window and are not limited to using the SharePoint Online Management Shell. (The -DisableNameChecking parameter of the Import-Module cmdlet simply tells PowerShell to not bother checking for valid verbs used by the loaded cmdlets and avoids warnings that are generated by the fact that the module does use an invalid verb – specifically, Upgrade). Note that unlike with the snap-in, there’s no need to specify the threading options because the cmdlets don’t use any unmanaged resources which need disposal.
Getting Connected
Now that you’ve got the SharePoint Online Management Shell installed you are now ready to connect to your tenant administration site. This initial connection is necessary to establish a connection context which stores the URL of the tenant administration site and the credentials used to connect to the site. To establish the connection use the Connect-SPOService cmdlet:
Connect-SPOService -Url https://contoso-admin.sharepoint.com -Credential gary@contoso.com
Running this cmdlet basically just stores a Microsoft.SharePoint.Client.ClientContext object in an internal static variable (or a sub-classed version of it at least). Future cmdlet calls then use this object to connect to the site, thereby negating the need to constantly provide the URL and credentials. (The downside of this object being internal is that we can’t extend the cmdlets to add our own, unless we want to use reflection which would be unsupported). To clear this internal variable (and make the session secure against other code that may attempt to use it) you can run the Disconnect-SPOService cmdlet. This cmdlet takes no parameters.
One tip to help make loading the module and then connecting to the site a tad bit easier would be to encapsulate the commands into a single helper method. In the following example I created a simple helper method named Connect-SPOSite which takes in the user and the tenant administration site to connect to, however, I default those values so that I only have to provide the password when I wish to get connected. I then put this method in my profile file (which you can edit by typing “ise $profile.CurrentUsersAllHosts”):
function Connect-SPOSite() {
param (
$user = "gary@contoso.com",
$site = "https://contoso-admin.sharepoint.com"
)
if ((Get-Module Microsoft.Online.SharePoint.PowerShell).Count -eq 0) {
Import-Module Microsoft.Online.SharePoint.PowerShell -DisableNameChecking
}
$cred = Get-Credential $user
Connect-SPOService -Url $site -Credential $cred
}
SPO Cmdlets
Now that you’re connected you can finally do something interesting. First let’s look at the cmdlets that are available. There are currently only 30 cmdlets available to us and you can see the list of those cmdlets by typing “Get-Command -Module Microsoft.Online.SharePoint.PowerShell”. Note that all of the cmdlets will have a noun which starts with “SPO”. The following is a list of all the available cmdlets:- Site Groups
- Get-SPOSiteGroup – Get a Site Collection Site Group.
- New-SPOSiteGroup – Create a new Site Collection Site Group.
- Remove-SPOSiteGroup – Remove an existing Site Collection Site Group.
- Set-SPOSiteGroup – Set the permission level and/or owner of an existing Site Collection Site Group.
- Users
- Add-SPOUser – Add a user to an existing Site Collection Site Group.
- Get-SPOUser – Get an existing user.
- Remove-SPOUser – Remove an existing user from the Site Collection or from an existing Site Collection Group.
- Set-SPOUser – Set whether an existing Site Collection user is a Site Collection administrator or not.
- Get-SPOExternalUser – Returns external users from the tenant’s folder.
- Remove-SPOExternalUser - Removes a collection of external users from the tenancy's folder.
- Site Collections
- Get-SPOSite – Retrieve an existing Site Collection.
- New-SPOSite – Create a new Site Collection.
- Remove-SPOSite – Move an existing Site Collection to the recycle bin.
- Repair-SPOSite – If any failed Site Collection scoped health check rules can perform an automatic repair then initiate the repair.
- Set-SPOSite – Set the Owner, Title, Storage Quota, Storage Quota Warning Level, Resource Quota, Resource Quota Warning Level, Locale ID, and/or whether the Site Collection allows Self Service Upgrade.
- Test-SPOSite – Run all Site Collection health check rules against the specified Site Collection.
- Upgrade-SPOSite – Upgrade the Site Collection. This can do a build-to-build (e.g., RTM to SP1) upgrade or a version-to-version (e.g., 2010 to 2013) upgrade. Use the -VersionUpgrade parameter for a version-to-version upgrade.
- Get-SPODeletedSite – Get a Site Collection from the recycle bin.
- Remove-SPODeletedSite – Remove a Site Collection from the recycle bin (permanently deletes it).
- Restore-SPODeletedSite – Restores an item from the recycle bin.
- Request-SPOUpgradeEvaluationSite - Creates a copy of the specified Site Collection and performs an upgrade on that copy.
- Get-SPOWebTemplate – Get a list of all available web templates.
- Tenants
- Get-SPOTenant – Retrieves information about the subscription tenant. This includes the Storage Quota size, Storage Quota Allocated (used), Resource Quota size, Resource Quota Allocated (used), Compatibility Range (14-14, 14-15, or 15-15), whether External Services are enabled, and the No Access Redirect URL.
- Get-SPOTenantLogEntry – Retrieves company logs (as of B2 only BCS logs are available).
- Get-SPOTenantLogLastAvailableTimeInUtc – Returns the time when the logs are collected.
- Set-SPOTenant – Sets the Minimum and Maximum Compatibility Level, whether External Services are enabled, and the No Access Redirect URL.
- Apps
- Get-SPOAppErrors – Returns application errors.
- Get-SPOAppInfo – Returns all installed applications.
- Connections
- Connect-SPOService – Establishes a connection to a SharePoint Online Tenant Administration Site.
- Disconnect-SPOService – Clears an existing connection.
The other thing to note is the lack of cmdlets for items at a lower scope than the Site Collection. Specifically there is no Get-SPOWeb or Get-SPOList cmdlet or anything of the sort. This can be potentially be quite limiting for most real world uses of PowerShell and, in my opinion, limit the usefulness of these new cmdlets to just the initial setup of a subscription and not the long-term maintenance of the subscription.
In the following examples I’ll walk through some examples of just a few of the more common cmdlets so that you can get an idea of the general usage of them.
Get a Site Collection
To see the list of Site Collections associated with a subscription or to see the details for a specific Site Collection use the Get-SPOSite cmdlet. This cmdlet has two parameter sets:Get-SPOSite [[-Identity] <SpoSitePipeBind>] [-Limit <string>] [-Detailed] [<CommonParameters>]
Get-SPOSite [-Filter <string>] [-Limit <string>] [-Detailed] [<CommonParameters>]
The parameter that you’ll want to pay the most attention to is the -Detailed parameter. If this optional switch parameter is omitted then the SPOSite objects that will be returned will only have their properties partially set. Now you might think that this is in order to reduce the traffic between the server and the client, however, all the properties are still sent over the wire, they simply have default values for everything other than a couple core properties (so I would assume the only performance improvement would be in the query on the server). You can see the difference in the values that are returned by looking at a Site Collection with and without the details:
PS C:\> Get-SPOSite https://contoso.sharepoint.com/ | select *
LastContentModifiedDate : 1/1/0001 12:00:00 AM
Status : Active
ResourceUsageCurrent : 0
ResourceUsageAverage : 0
StorageUsageCurrent : 0
LockIssue :
WebsCount : 0
CompatibilityLevel : 0
Url : https://contoso.sharepoint.com/
LocaleId : 1033
LockState : Unlock
Owner :
StorageQuota : 1000
StorageQuotaWarningLevel : 0
ResourceQuota : 300
ResourceQuotaWarningLevel : 255
Template : EHS#1
Title :
AllowSelfServiceUpgrade : False
PS C:\> Get-SPOSite https://contoso.sharepoint.com/ -Detailed | select *
LastContentModifiedDate : 11/2/2012 4:58:50 AM
Status : Active
ResourceUsageCurrent : 0
ResourceUsageAverage : 0
StorageUsageCurrent : 1
LockIssue :
WebsCount : 1
CompatibilityLevel : 15
Url : https://contoso.sharepoint.com/
LocaleId : 1033
LockState : Unlock
Owner : s-1-5-21-3176901541-3072848581-1985638908-189897
StorageQuota : 1000
StorageQuotaWarningLevel : 0
ResourceQuota : 300
ResourceQuotaWarningLevel : 255
Template : STS#0
Title : Contoso Team Site
AllowSelfServiceUpgrade : True
Create a Site Collection
When we’re ready to create a Site Collection we can use the New-SPOSite cmdlet. This cmdlet is very similar to the New-SPSite cmdlet that we have for on-premises deployments. The following shows the syntax for the cmdlet:New-SPOSite [-Url] <UrlCmdletPipeBind> -Owner <string> -StorageQuota <long> [-Title <string>] [-Template <string>] [-LocaleId <uint32>] [-CompatibilityLevel <int>] [-ResourceQuota <double>] [-TimeZoneId <int>] [-NoWait] [<CommonParameters>]
The following example demonstrates how we would call the cmdlet to create a new Site Collection called “Test”:
New-SPOSite -Url https://contoso.sharepoint.com/sites/Test -Title "Test" -Owner "gary@contoso.com" -Template "STS#0" -TimeZoneId 10 -StorageQuota 100
Note that the cmdlet also takes in a -NoWait parameter; this parameter tells the cmdlet to return immediately and not wait for the creation of the Site Collection to complete. If not specified then the cmdlet will poll the environment until it indicates that the Site Collection has been created. Using the -NoWait parameter is useful, however, when creating batches of Site Collections thereby allowing the operations to run asynchronously.
One issue you might bump into is in knowing which templates are available for your use. In the preceding example we are using the “STS#0” template, however, there are other templates available for our use and we can discover them using the Get-SPOWebTemplate cmdlet, as shown below:
PS C:\> Get-SPOWebTemplate
Name Title LocaleId CompatibilityLevel
---- ----- -------- ------------------
STS#0 Team Site 1033 15
BLOG#0 Blog 1033 15
BDR#0 Document Center 1033 15
DEV#0 Developer Site 1033 15
DOCMARKETPLACESITE#0 Academic Library 1033 15
OFFILE#1 Records Center 1033 15
EHS#1 Team Site - SharePoint Onl... 1033 15
BICenterSite#0 Business Intelligence Center 1033 15
SRCHCEN#0 Enterprise Search Center 1033 15
BLANKINTERNETCONTAINER#0 Publishing Portal 1033 15
ENTERWIKI#0 Enterprise Wiki 1033 15
PROJECTSITE#0 Project Site 1033 15
COMMUNITY#0 Community Site 1033 15
COMMUNITYPORTAL#0 Community Portal 1033 15
SRCHCENTERLITE#0 Basic Search Center 1033 15
visprus#0 Visio Process Repository 1033 15
Give Access to a Site Collection
Once your Site Collection has been created you may wish to grant users access to the Site Collection. First you may want to create a new SharePoint group (if an appropriate one is not already present) and then you may want to add users to that group (or an existing one). To accomplish these tasks you use the New-SPOSiteGroup cmdlet and the Add-SPOUser cmdlet, respectively.Looking at the New-SPOSiteGroup cmdlet you can see that it takes only three parameters, the name of the group to create, the permissions to add to the group, and the Site Collection within which to create the group:
New-SPOSiteGroup [-Site] <SpoSitePipeBind> [-Group] <string> [-PermissionLevels] <string[]> [<CommonParameters>]
In the following example I’m creating a new group named “Designers” and giving it the “Design” permission:
$site = Get-SPOSite https://contoso.sharepoint.com/sites/Test -Detailed
$group = New-SPOSiteGroup -Site $site -Group "Designers" -PermissionLevels "Design“
Once the group is created we can then use the Add-SPOUser cmdlet to add a user to the group. Like the New-SPOSiteGroup cmdlet this cmdlet takes three parameters:
Add-SPOUser [-Site] <SpoSitePipeBind> [-LoginName] <string> [-Group] <string> [<CommonParameters>]
In the following example I’m adding a new user to the previously created group:
Add-SPOUser -Site $site -Group $group.LoginName -LoginName "tessa@contoso.com"
Delete and Recover a Site Collection
If you’ve created a Site Collection that you now wish to delete you can easily accomplish this by using the Remove-SPOSite cmdlet. When this cmdlet finishes the Site Collection will have been moved to the recycle bin and not actually deleted. If you wish to permanently delete the Site Collection (and thus remove it from the recycle bin) then you must use the Remove-SPODeletedSite cmdlet. So to do a permanent delete it’s actually a two step process, as shown in the example below where I first move the “Test” Site Collection to the recycle bin and then delete it from the recycle bin:
Remove-SPOSite "http://contoso.sharepoint.com/sites/test" -Confirm:$false
Remove-SPODeletedSite -Identity "http://contoso.sharepoint.com/sites/test" -Confirm:$false
If you decide that you’d actually like to restore the Site Collection from the recycle bin you can simply use the Restore-SPODeletedSite cmdlet:
Restore-SPODeletedSite http://contoso.sharepoint.com/sites/test
Both the Remove-SPOSite and the Restore-SPODeletedSite cmdlets accept a -NoWait parameter which you can provide to tell the cmdlet to return immediately.
No comments:
Post a Comment